1752 lines
58 KiB
Julia
1752 lines
58 KiB
Julia
### A Pluto.jl notebook ###
|
||
# v0.19.12
|
||
|
||
using Markdown
|
||
using InteractiveUtils
|
||
|
||
# This Pluto notebook uses @bind for interactivity. When running this notebook outside of Pluto, the following 'mock version' of @bind gives bound variables a default value (instead of an error).
|
||
macro bind(def, element)
|
||
quote
|
||
local iv = try Base.loaded_modules[Base.PkgId(Base.UUID("6e696c72-6542-2067-7265-42206c756150"), "AbstractPlutoDingetjes")].Bonds.initial_value catch; b -> missing; end
|
||
local el = $(esc(element))
|
||
global $(esc(def)) = Core.applicable(Base.get, el) ? Base.get(el) : iv(el)
|
||
el
|
||
end
|
||
end
|
||
|
||
# ╔═╡ 96ec00fc-6f14-11eb-329e-19e4835643db
|
||
using PlutoUI; PlutoUI.TableOfContents(depth=2)
|
||
|
||
# ╔═╡ fec108ca-6f97-11eb-06d9-6fe1646f8b98
|
||
using Plots
|
||
|
||
# ╔═╡ 349d7534-6212-11eb-2bc5-db5be39b6bb6
|
||
md"""
|
||
# Numerical Methods of Medical Imaging - Exercise 1
|
||
|
||
[Institute for Biomedical Imaging](https://www.tuhh.de/ibi/home.html), Hamburg University of Technology
|
||
|
||
* 👨🏫 Prof. Dr. Tobias Knopp
|
||
* 👨🏫 Dr. Martin Möddel
|
||
|
||
###### Lab Course & Exercises
|
||
|
||
Exercises will cover numerical problems related to the lecture. It will be your task to solve these problems with the methods provided within the scope of the lecture and lab course. The lab course aims to teach you some core programming paradigms related to medical imaging and scientific programming.
|
||
"""
|
||
|
||
# ╔═╡ 6abc4d6a-da75-42bf-a62a-b98bb69585aa
|
||
md"""
|
||
# Getting Started with Julia
|
||
|
||
* 📅 Due date: 01.11.2022, 12 a.m.
|
||
* You can earn 0.5 point for exercise 1 to 10 and 1 point for the last exercise (6 points in total).
|
||
|
||
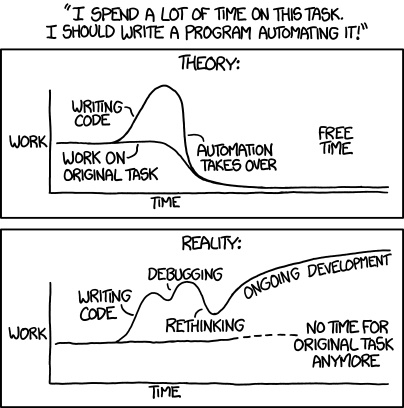
|
||
|
||
"Automation" taken from [xkcd](https://xkcd.com/1319/) is licensed under [CC](https://en.wikipedia.org/wiki/en:Creative_Commons) [BY-NC 2.5](https://creativecommons.org/licenses/by-nc/2.5/) license.
|
||
"""
|
||
|
||
# ╔═╡ 237ef27e-6266-11eb-3cf4-1b2223eabfd9
|
||
md"
|
||
## 1. Assignment Statements
|
||
|
||
An assignment statement creates a new variable and assigns a value to it. E.g.
|
||
"
|
||
|
||
# ╔═╡ 6c060eec-6266-11eb-0b23-e5be08d78823
|
||
text = "Hello World!"
|
||
|
||
# ╔═╡ 830c9ed0-6266-11eb-27ba-07773c842fed
|
||
md"""
|
||
assigns `"Hello World!"` to the variable `text`.
|
||
|
||
Variable names can be as long as you like. They can contain almost all Unicode characters, but must not begin with a number. It is allowed to use upper case letters, but it is common to use only lower case letters for variable names.
|
||
"""
|
||
|
||
# ╔═╡ f4270146-6216-11eb-391e-01a476fcfccd
|
||
md"
|
||
###### 🎓 a)
|
||
**Assign the number `10` to the Variable `n`.**
|
||
"
|
||
|
||
# ╔═╡ b5fff126-6215-11eb-1018-bd2e4f638f65
|
||
n = missing
|
||
|
||
# ╔═╡ 3249157e-6267-11eb-3dca-8949d7c0e3c9
|
||
md"
|
||
###### 🎓 b)
|
||
|
||
Unicode characters can be entered using the tab completion of $\mathrm{\LaTeX}$-like abbreviations.
|
||
|
||
**Assign a value to the Unicode character for the small alpha.**
|
||
"
|
||
|
||
# ╔═╡ ce1d05da-6267-11eb-136c-23c5c54a1559
|
||
😱 = 3
|
||
|
||
# ╔═╡ 1695a810-6268-11eb-3932-fb8885097f70
|
||
md"""
|
||
## 2. Arithmetic Operators
|
||
|
||
Arithmetic operations such as addition, subtraction, multiplication, division, and exponentiation can be performed by the operators `+`, `-`, `*`, `/`, and `^`, respectively. E.g.
|
||
"""
|
||
|
||
# ╔═╡ 77cefbd4-662e-11eb-1b1d-91da61cc3823
|
||
a1 = 2+2
|
||
|
||
# ╔═╡ 88776120-662e-11eb-1542-fd26e4f126b1
|
||
md"""
|
||
stores the result of $2+2$ in the variable `a1`. A full list of supported operations can be found [here](https://docs.julialang.org/en/v1/manual/mathematical-operations/).
|
||
"""
|
||
|
||
# ╔═╡ 874a1a5c-6632-11eb-2705-e914f01b9762
|
||
md"""
|
||
For mathematical operators, Julia follows mathematical conventions. Therefore, the following two expressions are equivalent.
|
||
"""
|
||
|
||
# ╔═╡ 812bbd7e-6632-11eb-29f8-3f48329f0ac9
|
||
2*a1
|
||
|
||
# ╔═╡ aeaa97ae-6632-11eb-0ea2-7febd8b3e965
|
||
2a1
|
||
|
||
# ╔═╡ b0d35a9a-662e-11eb-34f5-c9a5fd9bb9a6
|
||
md"
|
||
###### 🎓 a)
|
||
|
||
**Calculate $2^8$ with Julia and store the result in the variable `a2`.**
|
||
"
|
||
|
||
# ╔═╡ d285737a-662f-11eb-390e-1d1e2437de71
|
||
a2 = missing
|
||
|
||
# ╔═╡ 5d04cbea-6630-11eb-3bee-c182aa912653
|
||
md"
|
||
###### 🎓 b)
|
||
|
||
When an expression contains more than one operator, the order of evaluation depends on the operator precedence.
|
||
|
||
**Make use of parentheses `()` to group addition and exponentiation correctly to calculate $2^{4+4}$ with Julia and store the result in the variable `a3`.**
|
||
"
|
||
|
||
# ╔═╡ 0ae0cf56-6632-11eb-262a-191ea74ec517
|
||
a3 = missing
|
||
|
||
# ╔═╡ 0fe8c31e-663a-11eb-1acb-17d3d7615e64
|
||
md"""
|
||
## 3. Functions
|
||
|
||
In Julia, a function is an object that maps a tuple of argument values to a return value. They are not pure mathematical functions, because they can alter and be affected by the global state of the program.
|
||
|
||
The basic syntax for defining functions in Julia is:
|
||
"""
|
||
|
||
# ╔═╡ 478dde3c-663a-11eb-3244-e7449c93b3a5
|
||
function f(x,y)
|
||
return x + y
|
||
end
|
||
|
||
# ╔═╡ 68f2b1b0-663a-11eb-1b6d-b176d905f65b
|
||
md"
|
||
###### 🎓 a)
|
||
|
||
**Write a function `double(x)` that multiplies its input argument by 2.**
|
||
"
|
||
|
||
# ╔═╡ 8d509116-663b-11eb-0e98-dd27598740fe
|
||
function double(x)
|
||
return missing
|
||
end
|
||
|
||
# ╔═╡ 06c9bcec-663d-11eb-3062-85c0983a79eb
|
||
md"""
|
||
## 4. Conditional Evaluation
|
||
|
||
Conditional evaluation allows portions of code to be evaluated or not evaluated depending on the value of a boolean expression.
|
||
|
||
###### 🎓 a)
|
||
|
||
Read the julia documentation on [Conditional Evaluation](https://docs.julialang.org/en/v1/manual/control-flow/#man-conditional-evaluation).
|
||
|
||
**Define the Heaviside step function `heaviside(x)`, whose value is `0` for negative arguments and `1` for non-negative arguments.**
|
||
"""
|
||
|
||
# ╔═╡ 9fd96950-6651-11eb-25f7-c964ab504b4a
|
||
function heaviside(x)
|
||
return missing
|
||
end
|
||
|
||
# ╔═╡ 34824462-6654-11eb-2b38-19d14aa309af
|
||
md"""
|
||
## 5. Iteration
|
||
|
||
There are two constructs for repeated evaluation of expressions: the while loop and the for loop. Both are documented [here](https://docs.julialang.org/en/v1/manual/control-flow/#man-loops).
|
||
|
||
###### 🎓 a)
|
||
|
||
A prime number is only evenly divisible by itself and 1.
|
||
|
||
**Implement a function `isprime(x)` that returns `true` for any prime input and `false` else.**
|
||
"""
|
||
|
||
# ╔═╡ 6895356c-6655-11eb-3849-b3fa387df754
|
||
function isprime(x)
|
||
return missing
|
||
end
|
||
|
||
# ╔═╡ 9687bc24-666c-11eb-3b1e-edb5c448bad8
|
||
md"""
|
||
If you have trouble figuring out a solution you may find this hint helpful. However, first try solving the problem on your own!
|
||
"""
|
||
|
||
# ╔═╡ 9d3e9a92-6469-11eb-2952-b37367644c48
|
||
md"""
|
||
## 6. Primitive Numeric Types
|
||
|
||
In the documentation on [Integers and Floating-Point Numbers](https://docs.julialang.org/en/v1/manual/integers-and-floating-point-numbers/#Integers-and-Floating-Point-Numbers) we find:
|
||
|
||
> Julia provides a broad range of primitive numeric types, and a full complement of arithmetic and bitwise operators as well as standard mathematical functions are defined over them. These map directly onto numeric types and operations that are natively supported on modern computers, thus allowing Julia to take full advantage of computational resources.
|
||
|
||
You can inspect the type of any variable or value by using the `typeof` function
|
||
"""
|
||
|
||
# ╔═╡ f3cd5320-66d6-11eb-191c-4b4d8cba940d
|
||
typeof(1)
|
||
|
||
# ╔═╡ f5805956-66d6-11eb-04e8-b1faae8f0d3c
|
||
typeof(1.0)
|
||
|
||
# ╔═╡ c72359f4-66d7-11eb-395f-b3a1983a6eea
|
||
typeof(1+1.0)
|
||
|
||
# ╔═╡ bdea6774-66d7-11eb-1c62-8f0e935f98ef
|
||
md"""
|
||
###### 🎓 a)
|
||
Take a look into the documentation and assign an 8-bit unsigned integer of any value to the variable `m`.
|
||
"""
|
||
|
||
# ╔═╡ fb73ea0c-66d7-11eb-001c-23033aee228a
|
||
m = missing
|
||
|
||
# ╔═╡ 2b99da2c-666d-11eb-1c64-337654a9d8f2
|
||
md"""
|
||
## 7. Ranges
|
||
|
||
In Julia one can use range objects to represent a sequence of numbers. These can then be used to iterate through a loop. E.g.
|
||
"""
|
||
|
||
# ╔═╡ d39fbca0-66db-11eb-1aae-7b29f559cb01
|
||
for i in 1:5
|
||
continue # this does nothing and skips to the next iteration
|
||
end
|
||
|
||
# ╔═╡ 1bcd317c-66e8-11eb-10df-c132d5f79155
|
||
md"""
|
||
###### 🎓 a)
|
||
**Use a range object to sum up all values from `1` to `n` in the function `sumup(n)`.**
|
||
"""
|
||
|
||
# ╔═╡ 66c4f3fc-66db-11eb-0927-ebe1d40eeb3b
|
||
function sumup(n)
|
||
return missing
|
||
end
|
||
|
||
# ╔═╡ 575d9e52-6468-11eb-2f95-63cd3920f91a
|
||
md"""
|
||
## 8. Vectors
|
||
|
||
Often we want to store and process multiple values. In Julia one can combine a a sequence of values of any type into a vector.
|
||
|
||
There are several ways to create a new array, the simplest is to enclose the elements in square brackets.
|
||
"""
|
||
|
||
# ╔═╡ 2e6c3c30-66e6-11eb-10f0-ddaa1752cff9
|
||
["first element",2,3.0]
|
||
|
||
# ╔═╡ d701d778-66e7-11eb-16c2-ab49fc06e992
|
||
md"""
|
||
###### 🎓 a)
|
||
**Create a vector `v` containing the numbers 1 to 10 in ascending order.**
|
||
"""
|
||
|
||
# ╔═╡ 055baa32-66e7-11eb-20fc-575565bda51b
|
||
v = missing
|
||
|
||
# ╔═╡ 91c222ea-66e6-11eb-28ce-c1f1424525c8
|
||
md"""
|
||
## 9. Comprehensions
|
||
|
||
[Comprehensions](https://docs.julialang.org/en/v1/manual/arrays/#man-comprehensions) provide a general and powerful way to construct arrays. Comprehension syntax is similar to set construction notation in mathematics.
|
||
"""
|
||
|
||
# ╔═╡ b5db566a-66e6-11eb-35fb-17d3fc4e258c
|
||
A = [π*i for i in 1:5]
|
||
|
||
# ╔═╡ a44eb7ea-66e9-11eb-10fa-2b0936b9f489
|
||
md"""
|
||
###### 🎓 a)
|
||
**Create a vector `w` containing the numbers 2,4,6,... to 1000 in ascending order.**
|
||
"""
|
||
|
||
# ╔═╡ d8f306ca-66e9-11eb-3728-156d0328250b
|
||
w = missing
|
||
|
||
# ╔═╡ 673cc322-666e-11eb-107f-2b9bd6826ad5
|
||
md"""
|
||
## 10. Broadcasting
|
||
|
||
[Broadcasting](https://docs.julialang.org/en/v1/manual/arrays/#Broadcasting) enables the convenient vectorization of mathematical and other operations. To this end Julia provides the dot syntax, e.g.
|
||
"""
|
||
|
||
# ╔═╡ 1bf921be-66eb-11eb-089a-97dfe9418b32
|
||
sin.(A)
|
||
|
||
# ╔═╡ 455d0b7c-66eb-11eb-3167-4b204ac741a5
|
||
md"""
|
||
for element wise operations over arrays
|
||
"""
|
||
|
||
# ╔═╡ 529a7324-66eb-11eb-0c1f-c37639e37a6e
|
||
md"""
|
||
###### 🎓 a)
|
||
**Use the dot syntax to divide all elements of `A` by π and store the result in the variable `B`.**
|
||
"""
|
||
|
||
# ╔═╡ a87e36c4-66eb-11eb-223e-a1b077dca672
|
||
B = missing
|
||
|
||
# ╔═╡ 0aa99f86-6f97-11eb-2141-2d35c3e0857d
|
||
md"""
|
||
## 11. Julia Eco System
|
||
|
||
Julia has a wide ecosystem of packages, maintained by a wide variety of people. In the best of academic ideals, Julia users from across the world come together to create mutually compatible and supporting packages for their domains. To manage these collections of packages they often use GitHub organizations and various other communication channels, most also have channels on the main Julia Slack channel, and sub-forums on the main Julia Discourse forum (see [Community](https://discourse.julialang.org/)).
|
||
|
||
###### Pkg
|
||
Pkg is Julia's built-in package manager and handles operations such as installing, updating and removing packages.
|
||
|
||
Before we can start we need to add the packages we want to use. This can be done using the package manager `Pkg`. However Pluto has it's own build-in package manager and Pkg is not needed anymore if one works inside Pluto notebooks.
|
||
"""
|
||
|
||
# ╔═╡ f77039b0-6f97-11eb-177b-2730efcb4dcd
|
||
md"""
|
||
We will focus on how to make use of the `Plots` package.
|
||
|
||
We can start `using` methods and objects exported by packages by
|
||
"""
|
||
|
||
# ╔═╡ 1559f57e-6f98-11eb-3539-1b1ae82c439b
|
||
md"""
|
||
## 12. Plotting
|
||
|
||
[Plots](https://github.com/JuliaPlots/Plots.jl) is a visualization interface and tool set. It sits above other backends, like GR, PyPlot, PGFPlotsX, or Plotly, connecting commands with implementation. If one backend does not support your desired features or make the right trade-offs, you can just switch to another backend with one command. No need to change your code. No need to learn a new syntax.
|
||
|
||
The goals with the package are:
|
||
|
||
* Powerful. Do more with less. Complex visualizations become easy.
|
||
* Intuitive. Start generating plots without reading volumes of documentation. Commands should "just work."
|
||
* Concise. Less code means fewer mistakes and more efficient development and analysis.
|
||
* Consistent. Don't commit to one graphics package. Use the same code and access the strengths of all backends.
|
||
|
||
A `Plots` cheat sheet is available [here](https://github.com/sswatson/cheatsheets/blob/master/plotsjl-cheatsheet.pdf).
|
||
"""
|
||
|
||
# ╔═╡ 36e6783c-6f98-11eb-0b09-db56907e370d
|
||
md"""
|
||
Data is supplied to the `plot` function as arguments (`x`, or `x`,`y`, or `x`,`y`,`z`). To this end let us consider the following arguments
|
||
"""
|
||
|
||
# ╔═╡ 3f4422ce-6f98-11eb-111f-4d1624a326c7
|
||
begin
|
||
x = range(0,2π,length=100)
|
||
y = map(sin,x)
|
||
z = map(cos,x)
|
||
end;
|
||
|
||
# ╔═╡ 44ee1586-6f98-11eb-3452-f7db9e3738ad
|
||
md"""
|
||
###### 🎓 a)
|
||
**Call the plot function with a single argument. Try out all arguments `x`, `y`, and `z`.**
|
||
"""
|
||
|
||
# ╔═╡ 4a8f3088-6f98-11eb-1d0e-4b1ba2e676ae
|
||
missing
|
||
|
||
# ╔═╡ 50cc4f32-6f98-11eb-25a4-ebaf581955ea
|
||
md"""
|
||
###### 🎓 b)
|
||
**Call the plot function with two argument. Try out different combinations of arguments.**
|
||
"""
|
||
|
||
# ╔═╡ 56177258-6f98-11eb-276f-7d8053bdcb86
|
||
missing
|
||
|
||
# ╔═╡ 5733a026-6f98-11eb-1b50-c75f87fbabe5
|
||
md"""
|
||
###### 🎓 c)
|
||
**Call the plot function with all three arguments. What happens if you exchange their order?**
|
||
"""
|
||
|
||
# ╔═╡ 5d770eb4-6f98-11eb-3206-8d26f2717981
|
||
missing
|
||
|
||
# ╔═╡ 6824d1f2-6f98-11eb-12f1-adf1271af917
|
||
md"""
|
||
Arguments are interpreted flexible. We have already seen that we can plot `x`, which is no `Vector`, but an iterable object.
|
||
|
||
###### 🎓 d)
|
||
**Plot the `exp` function over the range given by `x` by passing `x` and `exp` directly.**
|
||
"""
|
||
|
||
# ╔═╡ 6debc444-6f98-11eb-3c9e-4dc533fe13ec
|
||
missing
|
||
|
||
# ╔═╡ 77d17b00-6f98-11eb-37ad-dd347db13fb3
|
||
md"""
|
||
Data can be plotted together as series, as is the default. There are different series types available to change the way the data is visualized.
|
||
|
||
###### 🎓 e)
|
||
**This can be achieved by using the `seriestype` keyword argument. Take your time and explore the different `seriestype` options listed.**
|
||
"""
|
||
|
||
# ╔═╡ 8b852fd4-6f98-11eb-1b3a-7ff47c51e99e
|
||
@bind seriestype1 Select(["line" => :line,"path" => :path, "steppre" => :steppre, "steppost" => :steppost, "sticks" => :sticks, "scatter" => :scatter])
|
||
|
||
# ╔═╡ 8f566768-6f98-11eb-20ae-45d6f39cd210
|
||
plot(y,z, seriestype=seriestype1)
|
||
|
||
# ╔═╡ 961e9cd2-6f98-11eb-362c-517edab85a8c
|
||
md"""
|
||
We can modify [attributes](https://docs.juliaplots.org/latest/attributes/) of a plot by passing keyword arguments (for example, `plot(y, color = :blue)`).
|
||
|
||
###### 🎓 f)
|
||
**Recreate the plot above with a label using the keyword `label`. Pass the `String` `"circle"` to the argument.**
|
||
"""
|
||
|
||
# ╔═╡ a32f2a4a-6f98-11eb-18f9-efb51aac288c
|
||
plot(y,z, label="circle")
|
||
|
||
# ╔═╡ acc530cc-6f98-11eb-330e-077fcaf5bd62
|
||
md"""
|
||
In most cases, passing a (`n` × `m`) matrix of values (numbers, etc) will create `m` series, each with `n` data points.
|
||
|
||
###### 🎓 g)
|
||
**Take your time and explore how the different options affect the plot.**
|
||
"""
|
||
|
||
# ╔═╡ e5ca1bf8-6f98-11eb-1bd9-6f2f1fbe55c9
|
||
# 100 data points in 4 series
|
||
yseries = [sin.(x) cos.(x) 2sin.(x) 2cos.(x)]
|
||
|
||
# ╔═╡ eba05204-6f98-11eb-1e7c-4f7b49962f23
|
||
@bind seriestype2 Select(["line" => :line,"path" => :path, "steppre" => :steppre, "steppost" => :steppost, "sticks" => :sticks, "scatter" => :scatter])
|
||
|
||
# ╔═╡ f22b7874-6f98-11eb-0417-1de0d171c4ad
|
||
plot(yseries,z, seriestype=seriestype2)
|
||
|
||
# ╔═╡ f8265ba4-6f98-11eb-3938-0b38b2b93285
|
||
md"""
|
||
We can pass attributes for each element in a series by passing a row vector
|
||
|
||
###### 🎓 h)
|
||
**Recreate the plot above with labels `["circle" "ellipse" "line1" "line2"]`.**
|
||
"""
|
||
|
||
# ╔═╡ fd8522ec-6f98-11eb-0a3c-01a91ee48de9
|
||
plot(yseries,z, seriestype=seriestype2, label = ["circle" "ellipse" "line1" "line2"])
|
||
|
||
# ╔═╡ 0cce95b2-6f99-11eb-1161-1d446c3bbe44
|
||
md"""
|
||
Using Plots we can also visualize rectangular data arrays using the heatmap function. This could be a map of temperatures, or population density for example.
|
||
|
||
Here a simple academic example of such an array
|
||
"""
|
||
|
||
# ╔═╡ 1a12d5a8-6f99-11eb-0e46-1529f881a3b0
|
||
begin
|
||
function pyramid(x,y)
|
||
u = abs(x)
|
||
v = abs(y)
|
||
return (1-max(u,v))
|
||
end
|
||
|
||
xs = -1.95:0.1:1.95
|
||
ys = -1.95:0.1:1.95
|
||
zs = [pyramid(x,y) for x in xs, y in ys]
|
||
end;
|
||
|
||
# ╔═╡ 214ce458-6f99-11eb-0963-7965b5fba93a
|
||
md"""
|
||
and its visualization
|
||
"""
|
||
|
||
# ╔═╡ 29efc990-6f99-11eb-0633-63fb74c5bebf
|
||
heatmap(xs,ys,zs)
|
||
|
||
# ╔═╡ 2f3b762e-6f99-11eb-12f0-2d42ed8237e0
|
||
md"""
|
||
Colorizing images helps the human visual system pick out detail, estimate quantitative values, and notice patterns in data in a more intuitive fashion. However, the choice of the color map can have a significant impact on a given task.
|
||
|
||
To this end `Plots` has a very large number of color maps available. A complete list of readily available schemes can be found [here](https://docs.juliaplots.org/latest/generated/colorschemes/).
|
||
|
||
###### 🎓 i)
|
||
**Explore the different color maps. Do they influence your perception of the linear rise flanks of the pyramid?**
|
||
"""
|
||
|
||
# ╔═╡ 372fa56c-6f99-11eb-115d-275699f1c05c
|
||
begin
|
||
colors = Dict("viridis" => :viridis, "blackbody" => :blackbody, "temperaturemap" => :temperaturemap, "thermometer" => :thermometer, "turbo" => :turbo, "vangogh" => :vangogh, "vermeer" => :vermeer, "pastel" => :pastel, "coffee" => :coffee);
|
||
@bind cname Select(collect(keys(colors)))
|
||
end
|
||
|
||
# ╔═╡ 473b6dec-6f99-11eb-04f0-07006f1996ba
|
||
heatmap(xs, ys, zs,
|
||
c = colors[cname],
|
||
xlims = (-2,2),
|
||
ylims = (-2,2),
|
||
aspect_ratio = 1,
|
||
xlabel = "x",
|
||
ylabel = "y"
|
||
)
|
||
|
||
# ╔═╡ bf493588-6f14-11eb-3ddf-b7ce036aff36
|
||
begin
|
||
hint(text) = Markdown.MD(Markdown.Admonition("hint", "Hint", [text]))
|
||
not_defined(variable_name) = Markdown.MD(Markdown.Admonition("danger", "Oh, oh! 😱", [md"Variable **$(Markdown.Code(string(variable_name)))** is not defined. You should probably do something about this."]))
|
||
still_missing(text=md"Replace `missing` with your solution.") = Markdown.MD(Markdown.Admonition("warning", "Let's go!", [text]))
|
||
keep_working(text=md"The answer is not quite right.") = Markdown.MD(Markdown.Admonition("danger", "Keep working on it!", [text]));
|
||
yays = [md"Great! 🥳", md"Correct! 👏", md"Tada! 🎉"]
|
||
correct(text=md"$(rand(yays)) Let's move on to the next task.") = Markdown.MD(Markdown.Admonition("correct", "Got it!", [text]))
|
||
end;
|
||
|
||
# ╔═╡ b9dbaf62-6215-11eb-3a7d-0b882b1a10b0
|
||
if !@isdefined(n)
|
||
not_defined(:n)
|
||
elseif ismissing(n)
|
||
still_missing()
|
||
elseif !(n isa Number)
|
||
keep_working(md"`n` has not been assigned a number.")
|
||
elseif n != 10
|
||
keep_working(md"`n` has been assigned the wrong value.")
|
||
else
|
||
correct()
|
||
end
|
||
|
||
# ╔═╡ cf933c04-6267-11eb-3317-ed1a42e8c64e
|
||
if !@isdefined(α)
|
||
not_defined(:α)
|
||
else
|
||
correct()
|
||
end
|
||
|
||
# ╔═╡ de2a045c-662f-11eb-1d80-65fe7d8e0db3
|
||
if !@isdefined(a2)
|
||
not_defined(:a2)
|
||
elseif ismissing(a2)
|
||
still_missing()
|
||
elseif !(a2 isa Number)
|
||
keep_working(md"`a2` has not been assigned a number.")
|
||
elseif a2 != 256
|
||
keep_working(md"`a2` has been assigned the wrong value.")
|
||
else
|
||
correct()
|
||
end
|
||
|
||
# ╔═╡ f279b1ee-6631-11eb-0809-bf0699c636f2
|
||
if !@isdefined(a3)
|
||
not_defined(:a3)
|
||
elseif ismissing(a3)
|
||
still_missing()
|
||
elseif !(a3 isa Number)
|
||
keep_working(md"`a3` has not been assigned a number.")
|
||
elseif a3 != 256
|
||
keep_working(md"`a3` has been assigned the wrong value.")
|
||
else
|
||
correct()
|
||
end
|
||
|
||
# ╔═╡ bb8694b8-663b-11eb-03a1-49713346bdf3
|
||
let x = rand()
|
||
if !@isdefined(double)
|
||
not_defined(:double)
|
||
elseif !hasmethod(double,Tuple{Int})
|
||
keep_working(md"No method `double` with a single input argument defined.")
|
||
elseif ismissing(double(0))
|
||
still_missing()
|
||
elseif double(0) !=0 || double(x) != 2x
|
||
keep_working(md"`double(x)` does not return twice its value.")
|
||
else
|
||
correct()
|
||
end
|
||
end
|
||
|
||
# ╔═╡ de2903cc-6652-11eb-30c3-7114b15fa6e1
|
||
if !@isdefined(heaviside)
|
||
not_defined(:heaviside)
|
||
elseif ismissing(heaviside(0))
|
||
still_missing()
|
||
elseif heaviside(0) !=1
|
||
keep_working(md"`heaviside(0)` does not return 1.")
|
||
elseif heaviside(-1) != 0
|
||
keep_working(md"`heaviside(-1)` does not return 0")
|
||
elseif heaviside(1) != 1
|
||
keep_working(md"`heaviside(0)` does not return 1.")
|
||
else
|
||
correct()
|
||
end
|
||
|
||
# ╔═╡ 6b9a4b64-6656-11eb-10ce-7b4a8b3cd6a4
|
||
if !@isdefined(isprime)
|
||
not_defined(:isprime)
|
||
elseif ismissing(isprime(1))
|
||
still_missing()
|
||
elseif typeof(isprime(1)) != Bool
|
||
keep_working(md"`isprime(1)` does return neither `true` or `false`.")
|
||
elseif isprime(1)
|
||
keep_working(md"`isprime(1)` does not return `false`.")
|
||
elseif !isprime(2)
|
||
keep_working(md"`isprime(2)` does not return `true`.")
|
||
elseif !isprime(2)
|
||
keep_working(md"`isprime(3)` does not return `true`.")
|
||
elseif isprime(4)
|
||
keep_working(md"`isprime(4)` does not return `false`.")
|
||
elseif !isprime(999331)
|
||
keep_working(md"`isprime(999331)` does not return `true`.")
|
||
else
|
||
correct()
|
||
end
|
||
|
||
# ╔═╡ a4a9afe4-6656-11eb-0664-83cb32ce934b
|
||
hint(md"Take a look at the documentations of the functions `rem` and `sqrt`. They might be helpful.")
|
||
|
||
# ╔═╡ 07491ca8-66d8-11eb-3304-99f911b4bd1d
|
||
if !@isdefined(m)
|
||
not_defined(:m)
|
||
elseif ismissing(m)
|
||
still_missing()
|
||
elseif !(m isa UInt8)
|
||
keep_working(md"`m` is not assigned a 8-bit unsigned integer.")
|
||
else
|
||
correct()
|
||
end
|
||
|
||
# ╔═╡ 2c63db0a-66dc-11eb-1a45-7902d591c3e1
|
||
if !@isdefined(sumup)
|
||
not_defined(:sumup)
|
||
elseif ismissing(sumup(0))
|
||
still_missing()
|
||
elseif sumup(0) != 0
|
||
keep_working(md"`sumup(0)` is expected to return 0.")
|
||
elseif sumup(100) != 5050
|
||
keep_working(md"`sumup(100)` is expected to return 5050.")
|
||
else
|
||
correct()
|
||
end
|
||
|
||
# ╔═╡ 6a3905f4-66e6-11eb-0607-5534821caee6
|
||
if !@isdefined(v)
|
||
not_defined(:v)
|
||
elseif ismissing(v)
|
||
still_missing()
|
||
elseif !(typeof(v) <: AbstractVector)
|
||
keep_working(md"`v` is no vector.")
|
||
elseif diff(v) != ones(9) || v[1] != 1
|
||
keep_working(md"`v` does not seem to contain the numbers 1 to 10.")
|
||
else
|
||
correct()
|
||
end
|
||
|
||
# ╔═╡ 2561d38a-66ea-11eb-10ab-27db1a87970b
|
||
if !@isdefined(w)
|
||
not_defined(:w)
|
||
elseif ismissing(w)
|
||
still_missing()
|
||
elseif !(typeof(w) <: AbstractVector)
|
||
keep_working(md"`w` is no vector.")
|
||
elseif diff(w) != 2*ones(499) || w[1] != 2
|
||
keep_working(md"`w` does not seem to contain the numbers 2,4,6,... to 1000.")
|
||
else
|
||
correct()
|
||
end
|
||
|
||
# ╔═╡ b135affc-66eb-11eb-188b-a32ef1478ee6
|
||
if !@isdefined(B)
|
||
not_defined(:B)
|
||
elseif ismissing(B)
|
||
still_missing()
|
||
elseif !(typeof(B) <: AbstractVector)
|
||
keep_working(md"`B` is no vector.")
|
||
elseif diff(B) != ones(4) || B[1] != 1
|
||
keep_working(md"`B` is expected to contain 1,2,3,4,5.")
|
||
else
|
||
correct()
|
||
end
|
||
|
||
# ╔═╡ 00000000-0000-0000-0000-000000000001
|
||
PLUTO_PROJECT_TOML_CONTENTS = """
|
||
[deps]
|
||
Plots = "91a5bcdd-55d7-5caf-9e0b-520d859cae80"
|
||
PlutoUI = "7f904dfe-b85e-4ff6-b463-dae2292396a8"
|
||
|
||
[compat]
|
||
Plots = "~1.35.2"
|
||
PlutoUI = "~0.7.43"
|
||
"""
|
||
|
||
# ╔═╡ 00000000-0000-0000-0000-000000000002
|
||
PLUTO_MANIFEST_TOML_CONTENTS = """
|
||
# This file is machine-generated - editing it directly is not advised
|
||
|
||
[[AbstractPlutoDingetjes]]
|
||
deps = ["Pkg"]
|
||
git-tree-sha1 = "8eaf9f1b4921132a4cff3f36a1d9ba923b14a481"
|
||
uuid = "6e696c72-6542-2067-7265-42206c756150"
|
||
version = "1.1.4"
|
||
|
||
[[ArgTools]]
|
||
uuid = "0dad84c5-d112-42e6-8d28-ef12dabb789f"
|
||
version = "1.1.1"
|
||
|
||
[[Artifacts]]
|
||
uuid = "56f22d72-fd6d-98f1-02f0-08ddc0907c33"
|
||
|
||
[[Base64]]
|
||
uuid = "2a0f44e3-6c83-55bd-87e4-b1978d98bd5f"
|
||
|
||
[[BitFlags]]
|
||
git-tree-sha1 = "84259bb6172806304b9101094a7cc4bc6f56dbc6"
|
||
uuid = "d1d4a3ce-64b1-5f1a-9ba4-7e7e69966f35"
|
||
version = "0.1.5"
|
||
|
||
[[Bzip2_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
|
||
git-tree-sha1 = "19a35467a82e236ff51bc17a3a44b69ef35185a2"
|
||
uuid = "6e34b625-4abd-537c-b88f-471c36dfa7a0"
|
||
version = "1.0.8+0"
|
||
|
||
[[Cairo_jll]]
|
||
deps = ["Artifacts", "Bzip2_jll", "Fontconfig_jll", "FreeType2_jll", "Glib_jll", "JLLWrappers", "LZO_jll", "Libdl", "Pixman_jll", "Pkg", "Xorg_libXext_jll", "Xorg_libXrender_jll", "Zlib_jll", "libpng_jll"]
|
||
git-tree-sha1 = "4b859a208b2397a7a623a03449e4636bdb17bcf2"
|
||
uuid = "83423d85-b0ee-5818-9007-b63ccbeb887a"
|
||
version = "1.16.1+1"
|
||
|
||
[[ChainRulesCore]]
|
||
deps = ["Compat", "LinearAlgebra", "SparseArrays"]
|
||
git-tree-sha1 = "e7ff6cadf743c098e08fca25c91103ee4303c9bb"
|
||
uuid = "d360d2e6-b24c-11e9-a2a3-2a2ae2dbcce4"
|
||
version = "1.15.6"
|
||
|
||
[[ChangesOfVariables]]
|
||
deps = ["ChainRulesCore", "LinearAlgebra", "Test"]
|
||
git-tree-sha1 = "38f7a08f19d8810338d4f5085211c7dfa5d5bdd8"
|
||
uuid = "9e997f8a-9a97-42d5-a9f1-ce6bfc15e2c0"
|
||
version = "0.1.4"
|
||
|
||
[[CodecZlib]]
|
||
deps = ["TranscodingStreams", "Zlib_jll"]
|
||
git-tree-sha1 = "ded953804d019afa9a3f98981d99b33e3db7b6da"
|
||
uuid = "944b1d66-785c-5afd-91f1-9de20f533193"
|
||
version = "0.7.0"
|
||
|
||
[[ColorSchemes]]
|
||
deps = ["ColorTypes", "ColorVectorSpace", "Colors", "FixedPointNumbers", "Random"]
|
||
git-tree-sha1 = "1fd869cc3875b57347f7027521f561cf46d1fcd8"
|
||
uuid = "35d6a980-a343-548e-a6ea-1d62b119f2f4"
|
||
version = "3.19.0"
|
||
|
||
[[ColorTypes]]
|
||
deps = ["FixedPointNumbers", "Random"]
|
||
git-tree-sha1 = "eb7f0f8307f71fac7c606984ea5fb2817275d6e4"
|
||
uuid = "3da002f7-5984-5a60-b8a6-cbb66c0b333f"
|
||
version = "0.11.4"
|
||
|
||
[[ColorVectorSpace]]
|
||
deps = ["ColorTypes", "FixedPointNumbers", "LinearAlgebra", "SpecialFunctions", "Statistics", "TensorCore"]
|
||
git-tree-sha1 = "d08c20eef1f2cbc6e60fd3612ac4340b89fea322"
|
||
uuid = "c3611d14-8923-5661-9e6a-0046d554d3a4"
|
||
version = "0.9.9"
|
||
|
||
[[Colors]]
|
||
deps = ["ColorTypes", "FixedPointNumbers", "Reexport"]
|
||
git-tree-sha1 = "417b0ed7b8b838aa6ca0a87aadf1bb9eb111ce40"
|
||
uuid = "5ae59095-9a9b-59fe-a467-6f913c188581"
|
||
version = "0.12.8"
|
||
|
||
[[Compat]]
|
||
deps = ["Dates", "LinearAlgebra", "UUIDs"]
|
||
git-tree-sha1 = "5856d3031cdb1f3b2b6340dfdc66b6d9a149a374"
|
||
uuid = "34da2185-b29b-5c13-b0c7-acf172513d20"
|
||
version = "4.2.0"
|
||
|
||
[[CompilerSupportLibraries_jll]]
|
||
deps = ["Artifacts", "Libdl"]
|
||
uuid = "e66e0078-7015-5450-92f7-15fbd957f2ae"
|
||
version = "0.5.2+0"
|
||
|
||
[[Contour]]
|
||
git-tree-sha1 = "d05d9e7b7aedff4e5b51a029dced05cfb6125781"
|
||
uuid = "d38c429a-6771-53c6-b99e-75d170b6e991"
|
||
version = "0.6.2"
|
||
|
||
[[DataAPI]]
|
||
git-tree-sha1 = "46d2680e618f8abd007bce0c3026cb0c4a8f2032"
|
||
uuid = "9a962f9c-6df0-11e9-0e5d-c546b8b5ee8a"
|
||
version = "1.12.0"
|
||
|
||
[[DataStructures]]
|
||
deps = ["Compat", "InteractiveUtils", "OrderedCollections"]
|
||
git-tree-sha1 = "d1fff3a548102f48987a52a2e0d114fa97d730f0"
|
||
uuid = "864edb3b-99cc-5e75-8d2d-829cb0a9cfe8"
|
||
version = "0.18.13"
|
||
|
||
[[Dates]]
|
||
deps = ["Printf"]
|
||
uuid = "ade2ca70-3891-5945-98fb-dc099432e06a"
|
||
|
||
[[DelimitedFiles]]
|
||
deps = ["Mmap"]
|
||
uuid = "8bb1440f-4735-579b-a4ab-409b98df4dab"
|
||
|
||
[[DocStringExtensions]]
|
||
deps = ["LibGit2"]
|
||
git-tree-sha1 = "5158c2b41018c5f7eb1470d558127ac274eca0c9"
|
||
uuid = "ffbed154-4ef7-542d-bbb7-c09d3a79fcae"
|
||
version = "0.9.1"
|
||
|
||
[[Downloads]]
|
||
deps = ["ArgTools", "FileWatching", "LibCURL", "NetworkOptions"]
|
||
uuid = "f43a241f-c20a-4ad4-852c-f6b1247861c6"
|
||
version = "1.6.0"
|
||
|
||
[[Expat_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
|
||
git-tree-sha1 = "bad72f730e9e91c08d9427d5e8db95478a3c323d"
|
||
uuid = "2e619515-83b5-522b-bb60-26c02a35a201"
|
||
version = "2.4.8+0"
|
||
|
||
[[FFMPEG]]
|
||
deps = ["FFMPEG_jll"]
|
||
git-tree-sha1 = "b57e3acbe22f8484b4b5ff66a7499717fe1a9cc8"
|
||
uuid = "c87230d0-a227-11e9-1b43-d7ebe4e7570a"
|
||
version = "0.4.1"
|
||
|
||
[[FFMPEG_jll]]
|
||
deps = ["Artifacts", "Bzip2_jll", "FreeType2_jll", "FriBidi_jll", "JLLWrappers", "LAME_jll", "Libdl", "Ogg_jll", "OpenSSL_jll", "Opus_jll", "PCRE2_jll", "Pkg", "Zlib_jll", "libaom_jll", "libass_jll", "libfdk_aac_jll", "libvorbis_jll", "x264_jll", "x265_jll"]
|
||
git-tree-sha1 = "74faea50c1d007c85837327f6775bea60b5492dd"
|
||
uuid = "b22a6f82-2f65-5046-a5b2-351ab43fb4e5"
|
||
version = "4.4.2+2"
|
||
|
||
[[FileWatching]]
|
||
uuid = "7b1f6079-737a-58dc-b8bc-7a2ca5c1b5ee"
|
||
|
||
[[FixedPointNumbers]]
|
||
deps = ["Statistics"]
|
||
git-tree-sha1 = "335bfdceacc84c5cdf16aadc768aa5ddfc5383cc"
|
||
uuid = "53c48c17-4a7d-5ca2-90c5-79b7896eea93"
|
||
version = "0.8.4"
|
||
|
||
[[Fontconfig_jll]]
|
||
deps = ["Artifacts", "Bzip2_jll", "Expat_jll", "FreeType2_jll", "JLLWrappers", "Libdl", "Libuuid_jll", "Pkg", "Zlib_jll"]
|
||
git-tree-sha1 = "21efd19106a55620a188615da6d3d06cd7f6ee03"
|
||
uuid = "a3f928ae-7b40-5064-980b-68af3947d34b"
|
||
version = "2.13.93+0"
|
||
|
||
[[Formatting]]
|
||
deps = ["Printf"]
|
||
git-tree-sha1 = "8339d61043228fdd3eb658d86c926cb282ae72a8"
|
||
uuid = "59287772-0a20-5a39-b81b-1366585eb4c0"
|
||
version = "0.4.2"
|
||
|
||
[[FreeType2_jll]]
|
||
deps = ["Artifacts", "Bzip2_jll", "JLLWrappers", "Libdl", "Pkg", "Zlib_jll"]
|
||
git-tree-sha1 = "87eb71354d8ec1a96d4a7636bd57a7347dde3ef9"
|
||
uuid = "d7e528f0-a631-5988-bf34-fe36492bcfd7"
|
||
version = "2.10.4+0"
|
||
|
||
[[FriBidi_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
|
||
git-tree-sha1 = "aa31987c2ba8704e23c6c8ba8a4f769d5d7e4f91"
|
||
uuid = "559328eb-81f9-559d-9380-de523a88c83c"
|
||
version = "1.0.10+0"
|
||
|
||
[[GLFW_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Libglvnd_jll", "Pkg", "Xorg_libXcursor_jll", "Xorg_libXi_jll", "Xorg_libXinerama_jll", "Xorg_libXrandr_jll"]
|
||
git-tree-sha1 = "d972031d28c8c8d9d7b41a536ad7bb0c2579caca"
|
||
uuid = "0656b61e-2033-5cc2-a64a-77c0f6c09b89"
|
||
version = "3.3.8+0"
|
||
|
||
[[GR]]
|
||
deps = ["Base64", "DelimitedFiles", "GR_jll", "HTTP", "JSON", "Libdl", "LinearAlgebra", "Pkg", "Preferences", "Printf", "Random", "Serialization", "Sockets", "Test", "UUIDs"]
|
||
git-tree-sha1 = "a9ec6a35bc5ddc3aeb8938f800dc599e652d0029"
|
||
uuid = "28b8d3ca-fb5f-59d9-8090-bfdbd6d07a71"
|
||
version = "0.69.3"
|
||
|
||
[[GR_jll]]
|
||
deps = ["Artifacts", "Bzip2_jll", "Cairo_jll", "FFMPEG_jll", "Fontconfig_jll", "GLFW_jll", "JLLWrappers", "JpegTurbo_jll", "Libdl", "Libtiff_jll", "Pixman_jll", "Pkg", "Qt5Base_jll", "Zlib_jll", "libpng_jll"]
|
||
git-tree-sha1 = "bc9f7725571ddb4ab2c4bc74fa397c1c5ad08943"
|
||
uuid = "d2c73de3-f751-5644-a686-071e5b155ba9"
|
||
version = "0.69.1+0"
|
||
|
||
[[Gettext_jll]]
|
||
deps = ["Artifacts", "CompilerSupportLibraries_jll", "JLLWrappers", "Libdl", "Libiconv_jll", "Pkg", "XML2_jll"]
|
||
git-tree-sha1 = "9b02998aba7bf074d14de89f9d37ca24a1a0b046"
|
||
uuid = "78b55507-aeef-58d4-861c-77aaff3498b1"
|
||
version = "0.21.0+0"
|
||
|
||
[[Glib_jll]]
|
||
deps = ["Artifacts", "Gettext_jll", "JLLWrappers", "Libdl", "Libffi_jll", "Libiconv_jll", "Libmount_jll", "PCRE2_jll", "Pkg", "Zlib_jll"]
|
||
git-tree-sha1 = "fb83fbe02fe57f2c068013aa94bcdf6760d3a7a7"
|
||
uuid = "7746bdde-850d-59dc-9ae8-88ece973131d"
|
||
version = "2.74.0+1"
|
||
|
||
[[Graphite2_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
|
||
git-tree-sha1 = "344bf40dcab1073aca04aa0df4fb092f920e4011"
|
||
uuid = "3b182d85-2403-5c21-9c21-1e1f0cc25472"
|
||
version = "1.3.14+0"
|
||
|
||
[[Grisu]]
|
||
git-tree-sha1 = "53bb909d1151e57e2484c3d1b53e19552b887fb2"
|
||
uuid = "42e2da0e-8278-4e71-bc24-59509adca0fe"
|
||
version = "1.0.2"
|
||
|
||
[[HTTP]]
|
||
deps = ["Base64", "CodecZlib", "Dates", "IniFile", "Logging", "LoggingExtras", "MbedTLS", "NetworkOptions", "OpenSSL", "Random", "SimpleBufferStream", "Sockets", "URIs", "UUIDs"]
|
||
git-tree-sha1 = "4abede886fcba15cd5fd041fef776b230d004cee"
|
||
uuid = "cd3eb016-35fb-5094-929b-558a96fad6f3"
|
||
version = "1.4.0"
|
||
|
||
[[HarfBuzz_jll]]
|
||
deps = ["Artifacts", "Cairo_jll", "Fontconfig_jll", "FreeType2_jll", "Glib_jll", "Graphite2_jll", "JLLWrappers", "Libdl", "Libffi_jll", "Pkg"]
|
||
git-tree-sha1 = "129acf094d168394e80ee1dc4bc06ec835e510a3"
|
||
uuid = "2e76f6c2-a576-52d4-95c1-20adfe4de566"
|
||
version = "2.8.1+1"
|
||
|
||
[[Hyperscript]]
|
||
deps = ["Test"]
|
||
git-tree-sha1 = "8d511d5b81240fc8e6802386302675bdf47737b9"
|
||
uuid = "47d2ed2b-36de-50cf-bf87-49c2cf4b8b91"
|
||
version = "0.0.4"
|
||
|
||
[[HypertextLiteral]]
|
||
deps = ["Tricks"]
|
||
git-tree-sha1 = "c47c5fa4c5308f27ccaac35504858d8914e102f9"
|
||
uuid = "ac1192a8-f4b3-4bfe-ba22-af5b92cd3ab2"
|
||
version = "0.9.4"
|
||
|
||
[[IOCapture]]
|
||
deps = ["Logging", "Random"]
|
||
git-tree-sha1 = "f7be53659ab06ddc986428d3a9dcc95f6fa6705a"
|
||
uuid = "b5f81e59-6552-4d32-b1f0-c071b021bf89"
|
||
version = "0.2.2"
|
||
|
||
[[IniFile]]
|
||
git-tree-sha1 = "f550e6e32074c939295eb5ea6de31849ac2c9625"
|
||
uuid = "83e8ac13-25f8-5344-8a64-a9f2b223428f"
|
||
version = "0.5.1"
|
||
|
||
[[InteractiveUtils]]
|
||
deps = ["Markdown"]
|
||
uuid = "b77e0a4c-d291-57a0-90e8-8db25a27a240"
|
||
|
||
[[InverseFunctions]]
|
||
deps = ["Test"]
|
||
git-tree-sha1 = "49510dfcb407e572524ba94aeae2fced1f3feb0f"
|
||
uuid = "3587e190-3f89-42d0-90ee-14403ec27112"
|
||
version = "0.1.8"
|
||
|
||
[[IrrationalConstants]]
|
||
git-tree-sha1 = "7fd44fd4ff43fc60815f8e764c0f352b83c49151"
|
||
uuid = "92d709cd-6900-40b7-9082-c6be49f344b6"
|
||
version = "0.1.1"
|
||
|
||
[[JLFzf]]
|
||
deps = ["Pipe", "REPL", "Random", "fzf_jll"]
|
||
git-tree-sha1 = "f377670cda23b6b7c1c0b3893e37451c5c1a2185"
|
||
uuid = "1019f520-868f-41f5-a6de-eb00f4b6a39c"
|
||
version = "0.1.5"
|
||
|
||
[[JLLWrappers]]
|
||
deps = ["Preferences"]
|
||
git-tree-sha1 = "abc9885a7ca2052a736a600f7fa66209f96506e1"
|
||
uuid = "692b3bcd-3c85-4b1f-b108-f13ce0eb3210"
|
||
version = "1.4.1"
|
||
|
||
[[JSON]]
|
||
deps = ["Dates", "Mmap", "Parsers", "Unicode"]
|
||
git-tree-sha1 = "3c837543ddb02250ef42f4738347454f95079d4e"
|
||
uuid = "682c06a0-de6a-54ab-a142-c8b1cf79cde6"
|
||
version = "0.21.3"
|
||
|
||
[[JpegTurbo_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
|
||
git-tree-sha1 = "b53380851c6e6664204efb2e62cd24fa5c47e4ba"
|
||
uuid = "aacddb02-875f-59d6-b918-886e6ef4fbf8"
|
||
version = "2.1.2+0"
|
||
|
||
[[LAME_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
|
||
git-tree-sha1 = "f6250b16881adf048549549fba48b1161acdac8c"
|
||
uuid = "c1c5ebd0-6772-5130-a774-d5fcae4a789d"
|
||
version = "3.100.1+0"
|
||
|
||
[[LERC_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
|
||
git-tree-sha1 = "bf36f528eec6634efc60d7ec062008f171071434"
|
||
uuid = "88015f11-f218-50d7-93a8-a6af411a945d"
|
||
version = "3.0.0+1"
|
||
|
||
[[LZO_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
|
||
git-tree-sha1 = "e5b909bcf985c5e2605737d2ce278ed791b89be6"
|
||
uuid = "dd4b983a-f0e5-5f8d-a1b7-129d4a5fb1ac"
|
||
version = "2.10.1+0"
|
||
|
||
[[LaTeXStrings]]
|
||
git-tree-sha1 = "f2355693d6778a178ade15952b7ac47a4ff97996"
|
||
uuid = "b964fa9f-0449-5b57-a5c2-d3ea65f4040f"
|
||
version = "1.3.0"
|
||
|
||
[[Latexify]]
|
||
deps = ["Formatting", "InteractiveUtils", "LaTeXStrings", "MacroTools", "Markdown", "OrderedCollections", "Printf", "Requires"]
|
||
git-tree-sha1 = "ab9aa169d2160129beb241cb2750ca499b4e90e9"
|
||
uuid = "23fbe1c1-3f47-55db-b15f-69d7ec21a316"
|
||
version = "0.15.17"
|
||
|
||
[[LibCURL]]
|
||
deps = ["LibCURL_jll", "MozillaCACerts_jll"]
|
||
uuid = "b27032c2-a3e7-50c8-80cd-2d36dbcbfd21"
|
||
version = "0.6.3"
|
||
|
||
[[LibCURL_jll]]
|
||
deps = ["Artifacts", "LibSSH2_jll", "Libdl", "MbedTLS_jll", "Zlib_jll", "nghttp2_jll"]
|
||
uuid = "deac9b47-8bc7-5906-a0fe-35ac56dc84c0"
|
||
version = "7.84.0+0"
|
||
|
||
[[LibGit2]]
|
||
deps = ["Base64", "NetworkOptions", "Printf", "SHA"]
|
||
uuid = "76f85450-5226-5b5a-8eaa-529ad045b433"
|
||
|
||
[[LibSSH2_jll]]
|
||
deps = ["Artifacts", "Libdl", "MbedTLS_jll"]
|
||
uuid = "29816b5a-b9ab-546f-933c-edad1886dfa8"
|
||
version = "1.10.2+0"
|
||
|
||
[[Libdl]]
|
||
uuid = "8f399da3-3557-5675-b5ff-fb832c97cbdb"
|
||
|
||
[[Libffi_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
|
||
git-tree-sha1 = "0b4a5d71f3e5200a7dff793393e09dfc2d874290"
|
||
uuid = "e9f186c6-92d2-5b65-8a66-fee21dc1b490"
|
||
version = "3.2.2+1"
|
||
|
||
[[Libgcrypt_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Libgpg_error_jll", "Pkg"]
|
||
git-tree-sha1 = "64613c82a59c120435c067c2b809fc61cf5166ae"
|
||
uuid = "d4300ac3-e22c-5743-9152-c294e39db1e4"
|
||
version = "1.8.7+0"
|
||
|
||
[[Libglvnd_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg", "Xorg_libX11_jll", "Xorg_libXext_jll"]
|
||
git-tree-sha1 = "7739f837d6447403596a75d19ed01fd08d6f56bf"
|
||
uuid = "7e76a0d4-f3c7-5321-8279-8d96eeed0f29"
|
||
version = "1.3.0+3"
|
||
|
||
[[Libgpg_error_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
|
||
git-tree-sha1 = "c333716e46366857753e273ce6a69ee0945a6db9"
|
||
uuid = "7add5ba3-2f88-524e-9cd5-f83b8a55f7b8"
|
||
version = "1.42.0+0"
|
||
|
||
[[Libiconv_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
|
||
git-tree-sha1 = "42b62845d70a619f063a7da093d995ec8e15e778"
|
||
uuid = "94ce4f54-9a6c-5748-9c1c-f9c7231a4531"
|
||
version = "1.16.1+1"
|
||
|
||
[[Libmount_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
|
||
git-tree-sha1 = "9c30530bf0effd46e15e0fdcf2b8636e78cbbd73"
|
||
uuid = "4b2f31a3-9ecc-558c-b454-b3730dcb73e9"
|
||
version = "2.35.0+0"
|
||
|
||
[[Libtiff_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "JpegTurbo_jll", "LERC_jll", "Libdl", "Pkg", "Zlib_jll", "Zstd_jll"]
|
||
git-tree-sha1 = "3eb79b0ca5764d4799c06699573fd8f533259713"
|
||
uuid = "89763e89-9b03-5906-acba-b20f662cd828"
|
||
version = "4.4.0+0"
|
||
|
||
[[Libuuid_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
|
||
git-tree-sha1 = "7f3efec06033682db852f8b3bc3c1d2b0a0ab066"
|
||
uuid = "38a345b3-de98-5d2b-a5d3-14cd9215e700"
|
||
version = "2.36.0+0"
|
||
|
||
[[LinearAlgebra]]
|
||
deps = ["Libdl", "libblastrampoline_jll"]
|
||
uuid = "37e2e46d-f89d-539d-b4ee-838fcccc9c8e"
|
||
|
||
[[LogExpFunctions]]
|
||
deps = ["ChainRulesCore", "ChangesOfVariables", "DocStringExtensions", "InverseFunctions", "IrrationalConstants", "LinearAlgebra"]
|
||
git-tree-sha1 = "94d9c52ca447e23eac0c0f074effbcd38830deb5"
|
||
uuid = "2ab3a3ac-af41-5b50-aa03-7779005ae688"
|
||
version = "0.3.18"
|
||
|
||
[[Logging]]
|
||
uuid = "56ddb016-857b-54e1-b83d-db4d58db5568"
|
||
|
||
[[LoggingExtras]]
|
||
deps = ["Dates", "Logging"]
|
||
git-tree-sha1 = "5d4d2d9904227b8bd66386c1138cf4d5ffa826bf"
|
||
uuid = "e6f89c97-d47a-5376-807f-9c37f3926c36"
|
||
version = "0.4.9"
|
||
|
||
[[MacroTools]]
|
||
deps = ["Markdown", "Random"]
|
||
git-tree-sha1 = "42324d08725e200c23d4dfb549e0d5d89dede2d2"
|
||
uuid = "1914dd2f-81c6-5fcd-8719-6d5c9610ff09"
|
||
version = "0.5.10"
|
||
|
||
[[Markdown]]
|
||
deps = ["Base64"]
|
||
uuid = "d6f4376e-aef5-505a-96c1-9c027394607a"
|
||
|
||
[[MbedTLS]]
|
||
deps = ["Dates", "MbedTLS_jll", "MozillaCACerts_jll", "Random", "Sockets"]
|
||
git-tree-sha1 = "6872f9594ff273da6d13c7c1a1545d5a8c7d0c1c"
|
||
uuid = "739be429-bea8-5141-9913-cc70e7f3736d"
|
||
version = "1.1.6"
|
||
|
||
[[MbedTLS_jll]]
|
||
deps = ["Artifacts", "Libdl"]
|
||
uuid = "c8ffd9c3-330d-5841-b78e-0817d7145fa1"
|
||
version = "2.28.0+0"
|
||
|
||
[[Measures]]
|
||
git-tree-sha1 = "e498ddeee6f9fdb4551ce855a46f54dbd900245f"
|
||
uuid = "442fdcdd-2543-5da2-b0f3-8c86c306513e"
|
||
version = "0.3.1"
|
||
|
||
[[Missings]]
|
||
deps = ["DataAPI"]
|
||
git-tree-sha1 = "bf210ce90b6c9eed32d25dbcae1ebc565df2687f"
|
||
uuid = "e1d29d7a-bbdc-5cf2-9ac0-f12de2c33e28"
|
||
version = "1.0.2"
|
||
|
||
[[Mmap]]
|
||
uuid = "a63ad114-7e13-5084-954f-fe012c677804"
|
||
|
||
[[MozillaCACerts_jll]]
|
||
uuid = "14a3606d-f60d-562e-9121-12d972cd8159"
|
||
version = "2022.2.1"
|
||
|
||
[[NaNMath]]
|
||
deps = ["OpenLibm_jll"]
|
||
git-tree-sha1 = "a7c3d1da1189a1c2fe843a3bfa04d18d20eb3211"
|
||
uuid = "77ba4419-2d1f-58cd-9bb1-8ffee604a2e3"
|
||
version = "1.0.1"
|
||
|
||
[[NetworkOptions]]
|
||
uuid = "ca575930-c2e3-43a9-ace4-1e988b2c1908"
|
||
version = "1.2.0"
|
||
|
||
[[Ogg_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
|
||
git-tree-sha1 = "887579a3eb005446d514ab7aeac5d1d027658b8f"
|
||
uuid = "e7412a2a-1a6e-54c0-be00-318e2571c051"
|
||
version = "1.3.5+1"
|
||
|
||
[[OpenBLAS_jll]]
|
||
deps = ["Artifacts", "CompilerSupportLibraries_jll", "Libdl"]
|
||
uuid = "4536629a-c528-5b80-bd46-f80d51c5b363"
|
||
version = "0.3.20+0"
|
||
|
||
[[OpenLibm_jll]]
|
||
deps = ["Artifacts", "Libdl"]
|
||
uuid = "05823500-19ac-5b8b-9628-191a04bc5112"
|
||
version = "0.8.1+0"
|
||
|
||
[[OpenSSL]]
|
||
deps = ["BitFlags", "Dates", "MozillaCACerts_jll", "OpenSSL_jll", "Sockets"]
|
||
git-tree-sha1 = "ebe81469e9d7b471d7ddb611d9e147ea16de0add"
|
||
uuid = "4d8831e6-92b7-49fb-bdf8-b643e874388c"
|
||
version = "1.2.1"
|
||
|
||
[[OpenSSL_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
|
||
git-tree-sha1 = "e60321e3f2616584ff98f0a4f18d98ae6f89bbb3"
|
||
uuid = "458c3c95-2e84-50aa-8efc-19380b2a3a95"
|
||
version = "1.1.17+0"
|
||
|
||
[[OpenSpecFun_jll]]
|
||
deps = ["Artifacts", "CompilerSupportLibraries_jll", "JLLWrappers", "Libdl", "Pkg"]
|
||
git-tree-sha1 = "13652491f6856acfd2db29360e1bbcd4565d04f1"
|
||
uuid = "efe28fd5-8261-553b-a9e1-b2916fc3738e"
|
||
version = "0.5.5+0"
|
||
|
||
[[Opus_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
|
||
git-tree-sha1 = "51a08fb14ec28da2ec7a927c4337e4332c2a4720"
|
||
uuid = "91d4177d-7536-5919-b921-800302f37372"
|
||
version = "1.3.2+0"
|
||
|
||
[[OrderedCollections]]
|
||
git-tree-sha1 = "85f8e6578bf1f9ee0d11e7bb1b1456435479d47c"
|
||
uuid = "bac558e1-5e72-5ebc-8fee-abe8a469f55d"
|
||
version = "1.4.1"
|
||
|
||
[[PCRE2_jll]]
|
||
deps = ["Artifacts", "Libdl"]
|
||
uuid = "efcefdf7-47ab-520b-bdef-62a2eaa19f15"
|
||
version = "10.40.0+0"
|
||
|
||
[[Parsers]]
|
||
deps = ["Dates"]
|
||
git-tree-sha1 = "3d5bf43e3e8b412656404ed9466f1dcbf7c50269"
|
||
uuid = "69de0a69-1ddd-5017-9359-2bf0b02dc9f0"
|
||
version = "2.4.0"
|
||
|
||
[[Pipe]]
|
||
git-tree-sha1 = "6842804e7867b115ca9de748a0cf6b364523c16d"
|
||
uuid = "b98c9c47-44ae-5843-9183-064241ee97a0"
|
||
version = "1.3.0"
|
||
|
||
[[Pixman_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
|
||
git-tree-sha1 = "b4f5d02549a10e20780a24fce72bea96b6329e29"
|
||
uuid = "30392449-352a-5448-841d-b1acce4e97dc"
|
||
version = "0.40.1+0"
|
||
|
||
[[Pkg]]
|
||
deps = ["Artifacts", "Dates", "Downloads", "LibGit2", "Libdl", "Logging", "Markdown", "Printf", "REPL", "Random", "SHA", "Serialization", "TOML", "Tar", "UUIDs", "p7zip_jll"]
|
||
uuid = "44cfe95a-1eb2-52ea-b672-e2afdf69b78f"
|
||
version = "1.8.0"
|
||
|
||
[[PlotThemes]]
|
||
deps = ["PlotUtils", "Statistics"]
|
||
git-tree-sha1 = "8162b2f8547bc23876edd0c5181b27702ae58dce"
|
||
uuid = "ccf2f8ad-2431-5c83-bf29-c5338b663b6a"
|
||
version = "3.0.0"
|
||
|
||
[[PlotUtils]]
|
||
deps = ["ColorSchemes", "Colors", "Dates", "Printf", "Random", "Reexport", "SnoopPrecompile", "Statistics"]
|
||
git-tree-sha1 = "21303256d239f6b484977314674aef4bb1fe4420"
|
||
uuid = "995b91a9-d308-5afd-9ec6-746e21dbc043"
|
||
version = "1.3.1"
|
||
|
||
[[Plots]]
|
||
deps = ["Base64", "Contour", "Dates", "Downloads", "FFMPEG", "FixedPointNumbers", "GR", "JLFzf", "JSON", "LaTeXStrings", "Latexify", "LinearAlgebra", "Measures", "NaNMath", "Pkg", "PlotThemes", "PlotUtils", "Printf", "REPL", "Random", "RecipesBase", "RecipesPipeline", "Reexport", "RelocatableFolders", "Requires", "Scratch", "Showoff", "SnoopPrecompile", "SparseArrays", "Statistics", "StatsBase", "UUIDs", "UnicodeFun", "Unzip"]
|
||
git-tree-sha1 = "65451f70d8d71bd9d06821c7a53adbed162454c9"
|
||
uuid = "91a5bcdd-55d7-5caf-9e0b-520d859cae80"
|
||
version = "1.35.2"
|
||
|
||
[[PlutoUI]]
|
||
deps = ["AbstractPlutoDingetjes", "Base64", "ColorTypes", "Dates", "Hyperscript", "HypertextLiteral", "IOCapture", "InteractiveUtils", "JSON", "Logging", "Markdown", "Random", "Reexport", "UUIDs"]
|
||
git-tree-sha1 = "2777a5c2c91b3145f5aa75b61bb4c2eb38797136"
|
||
uuid = "7f904dfe-b85e-4ff6-b463-dae2292396a8"
|
||
version = "0.7.43"
|
||
|
||
[[Preferences]]
|
||
deps = ["TOML"]
|
||
git-tree-sha1 = "47e5f437cc0e7ef2ce8406ce1e7e24d44915f88d"
|
||
uuid = "21216c6a-2e73-6563-6e65-726566657250"
|
||
version = "1.3.0"
|
||
|
||
[[Printf]]
|
||
deps = ["Unicode"]
|
||
uuid = "de0858da-6303-5e67-8744-51eddeeeb8d7"
|
||
|
||
[[Qt5Base_jll]]
|
||
deps = ["Artifacts", "CompilerSupportLibraries_jll", "Fontconfig_jll", "Glib_jll", "JLLWrappers", "Libdl", "Libglvnd_jll", "OpenSSL_jll", "Pkg", "Xorg_libXext_jll", "Xorg_libxcb_jll", "Xorg_xcb_util_image_jll", "Xorg_xcb_util_keysyms_jll", "Xorg_xcb_util_renderutil_jll", "Xorg_xcb_util_wm_jll", "Zlib_jll", "xkbcommon_jll"]
|
||
git-tree-sha1 = "c6c0f690d0cc7caddb74cef7aa847b824a16b256"
|
||
uuid = "ea2cea3b-5b76-57ae-a6ef-0a8af62496e1"
|
||
version = "5.15.3+1"
|
||
|
||
[[REPL]]
|
||
deps = ["InteractiveUtils", "Markdown", "Sockets", "Unicode"]
|
||
uuid = "3fa0cd96-eef1-5676-8a61-b3b8758bbffb"
|
||
|
||
[[Random]]
|
||
deps = ["SHA", "Serialization"]
|
||
uuid = "9a3f8284-a2c9-5f02-9a11-845980a1fd5c"
|
||
|
||
[[RecipesBase]]
|
||
deps = ["SnoopPrecompile"]
|
||
git-tree-sha1 = "612a4d76ad98e9722c8ba387614539155a59e30c"
|
||
uuid = "3cdcf5f2-1ef4-517c-9805-6587b60abb01"
|
||
version = "1.3.0"
|
||
|
||
[[RecipesPipeline]]
|
||
deps = ["Dates", "NaNMath", "PlotUtils", "RecipesBase"]
|
||
git-tree-sha1 = "017f217e647cf20b0081b9be938b78c3443356a0"
|
||
uuid = "01d81517-befc-4cb6-b9ec-a95719d0359c"
|
||
version = "0.6.6"
|
||
|
||
[[Reexport]]
|
||
git-tree-sha1 = "45e428421666073eab6f2da5c9d310d99bb12f9b"
|
||
uuid = "189a3867-3050-52da-a836-e630ba90ab69"
|
||
version = "1.2.2"
|
||
|
||
[[RelocatableFolders]]
|
||
deps = ["SHA", "Scratch"]
|
||
git-tree-sha1 = "90bc7a7c96410424509e4263e277e43250c05691"
|
||
uuid = "05181044-ff0b-4ac5-8273-598c1e38db00"
|
||
version = "1.0.0"
|
||
|
||
[[Requires]]
|
||
deps = ["UUIDs"]
|
||
git-tree-sha1 = "838a3a4188e2ded87a4f9f184b4b0d78a1e91cb7"
|
||
uuid = "ae029012-a4dd-5104-9daa-d747884805df"
|
||
version = "1.3.0"
|
||
|
||
[[SHA]]
|
||
uuid = "ea8e919c-243c-51af-8825-aaa63cd721ce"
|
||
version = "0.7.0"
|
||
|
||
[[Scratch]]
|
||
deps = ["Dates"]
|
||
git-tree-sha1 = "f94f779c94e58bf9ea243e77a37e16d9de9126bd"
|
||
uuid = "6c6a2e73-6563-6170-7368-637461726353"
|
||
version = "1.1.1"
|
||
|
||
[[Serialization]]
|
||
uuid = "9e88b42a-f829-5b0c-bbe9-9e923198166b"
|
||
|
||
[[Showoff]]
|
||
deps = ["Dates", "Grisu"]
|
||
git-tree-sha1 = "91eddf657aca81df9ae6ceb20b959ae5653ad1de"
|
||
uuid = "992d4aef-0814-514b-bc4d-f2e9a6c4116f"
|
||
version = "1.0.3"
|
||
|
||
[[SimpleBufferStream]]
|
||
git-tree-sha1 = "874e8867b33a00e784c8a7e4b60afe9e037b74e1"
|
||
uuid = "777ac1f9-54b0-4bf8-805c-2214025038e7"
|
||
version = "1.1.0"
|
||
|
||
[[SnoopPrecompile]]
|
||
git-tree-sha1 = "f604441450a3c0569830946e5b33b78c928e1a85"
|
||
uuid = "66db9d55-30c0-4569-8b51-7e840670fc0c"
|
||
version = "1.0.1"
|
||
|
||
[[Sockets]]
|
||
uuid = "6462fe0b-24de-5631-8697-dd941f90decc"
|
||
|
||
[[SortingAlgorithms]]
|
||
deps = ["DataStructures"]
|
||
git-tree-sha1 = "b3363d7460f7d098ca0912c69b082f75625d7508"
|
||
uuid = "a2af1166-a08f-5f64-846c-94a0d3cef48c"
|
||
version = "1.0.1"
|
||
|
||
[[SparseArrays]]
|
||
deps = ["LinearAlgebra", "Random"]
|
||
uuid = "2f01184e-e22b-5df5-ae63-d93ebab69eaf"
|
||
|
||
[[SpecialFunctions]]
|
||
deps = ["ChainRulesCore", "IrrationalConstants", "LogExpFunctions", "OpenLibm_jll", "OpenSpecFun_jll"]
|
||
git-tree-sha1 = "d75bda01f8c31ebb72df80a46c88b25d1c79c56d"
|
||
uuid = "276daf66-3868-5448-9aa4-cd146d93841b"
|
||
version = "2.1.7"
|
||
|
||
[[Statistics]]
|
||
deps = ["LinearAlgebra", "SparseArrays"]
|
||
uuid = "10745b16-79ce-11e8-11f9-7d13ad32a3b2"
|
||
|
||
[[StatsAPI]]
|
||
deps = ["LinearAlgebra"]
|
||
git-tree-sha1 = "f9af7f195fb13589dd2e2d57fdb401717d2eb1f6"
|
||
uuid = "82ae8749-77ed-4fe6-ae5f-f523153014b0"
|
||
version = "1.5.0"
|
||
|
||
[[StatsBase]]
|
||
deps = ["DataAPI", "DataStructures", "LinearAlgebra", "LogExpFunctions", "Missings", "Printf", "Random", "SortingAlgorithms", "SparseArrays", "Statistics", "StatsAPI"]
|
||
git-tree-sha1 = "d1bf48bfcc554a3761a133fe3a9bb01488e06916"
|
||
uuid = "2913bbd2-ae8a-5f71-8c99-4fb6c76f3a91"
|
||
version = "0.33.21"
|
||
|
||
[[TOML]]
|
||
deps = ["Dates"]
|
||
uuid = "fa267f1f-6049-4f14-aa54-33bafae1ed76"
|
||
version = "1.0.0"
|
||
|
||
[[Tar]]
|
||
deps = ["ArgTools", "SHA"]
|
||
uuid = "a4e569a6-e804-4fa4-b0f3-eef7a1d5b13e"
|
||
version = "1.10.1"
|
||
|
||
[[TensorCore]]
|
||
deps = ["LinearAlgebra"]
|
||
git-tree-sha1 = "1feb45f88d133a655e001435632f019a9a1bcdb6"
|
||
uuid = "62fd8b95-f654-4bbd-a8a5-9c27f68ccd50"
|
||
version = "0.1.1"
|
||
|
||
[[Test]]
|
||
deps = ["InteractiveUtils", "Logging", "Random", "Serialization"]
|
||
uuid = "8dfed614-e22c-5e08-85e1-65c5234f0b40"
|
||
|
||
[[TranscodingStreams]]
|
||
deps = ["Random", "Test"]
|
||
git-tree-sha1 = "8a75929dcd3c38611db2f8d08546decb514fcadf"
|
||
uuid = "3bb67fe8-82b1-5028-8e26-92a6c54297fa"
|
||
version = "0.9.9"
|
||
|
||
[[Tricks]]
|
||
git-tree-sha1 = "6bac775f2d42a611cdfcd1fb217ee719630c4175"
|
||
uuid = "410a4b4d-49e4-4fbc-ab6d-cb71b17b3775"
|
||
version = "0.1.6"
|
||
|
||
[[URIs]]
|
||
git-tree-sha1 = "e59ecc5a41b000fa94423a578d29290c7266fc10"
|
||
uuid = "5c2747f8-b7ea-4ff2-ba2e-563bfd36b1d4"
|
||
version = "1.4.0"
|
||
|
||
[[UUIDs]]
|
||
deps = ["Random", "SHA"]
|
||
uuid = "cf7118a7-6976-5b1a-9a39-7adc72f591a4"
|
||
|
||
[[Unicode]]
|
||
uuid = "4ec0a83e-493e-50e2-b9ac-8f72acf5a8f5"
|
||
|
||
[[UnicodeFun]]
|
||
deps = ["REPL"]
|
||
git-tree-sha1 = "53915e50200959667e78a92a418594b428dffddf"
|
||
uuid = "1cfade01-22cf-5700-b092-accc4b62d6e1"
|
||
version = "0.4.1"
|
||
|
||
[[Unzip]]
|
||
git-tree-sha1 = "ca0969166a028236229f63514992fc073799bb78"
|
||
uuid = "41fe7b60-77ed-43a1-b4f0-825fd5a5650d"
|
||
version = "0.2.0"
|
||
|
||
[[Wayland_jll]]
|
||
deps = ["Artifacts", "Expat_jll", "JLLWrappers", "Libdl", "Libffi_jll", "Pkg", "XML2_jll"]
|
||
git-tree-sha1 = "3e61f0b86f90dacb0bc0e73a0c5a83f6a8636e23"
|
||
uuid = "a2964d1f-97da-50d4-b82a-358c7fce9d89"
|
||
version = "1.19.0+0"
|
||
|
||
[[Wayland_protocols_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
|
||
git-tree-sha1 = "4528479aa01ee1b3b4cd0e6faef0e04cf16466da"
|
||
uuid = "2381bf8a-dfd0-557d-9999-79630e7b1b91"
|
||
version = "1.25.0+0"
|
||
|
||
[[XML2_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Libiconv_jll", "Pkg", "Zlib_jll"]
|
||
git-tree-sha1 = "58443b63fb7e465a8a7210828c91c08b92132dff"
|
||
uuid = "02c8fc9c-b97f-50b9-bbe4-9be30ff0a78a"
|
||
version = "2.9.14+0"
|
||
|
||
[[XSLT_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Libgcrypt_jll", "Libgpg_error_jll", "Libiconv_jll", "Pkg", "XML2_jll", "Zlib_jll"]
|
||
git-tree-sha1 = "91844873c4085240b95e795f692c4cec4d805f8a"
|
||
uuid = "aed1982a-8fda-507f-9586-7b0439959a61"
|
||
version = "1.1.34+0"
|
||
|
||
[[Xorg_libX11_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg", "Xorg_libxcb_jll", "Xorg_xtrans_jll"]
|
||
git-tree-sha1 = "5be649d550f3f4b95308bf0183b82e2582876527"
|
||
uuid = "4f6342f7-b3d2-589e-9d20-edeb45f2b2bc"
|
||
version = "1.6.9+4"
|
||
|
||
[[Xorg_libXau_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
|
||
git-tree-sha1 = "4e490d5c960c314f33885790ed410ff3a94ce67e"
|
||
uuid = "0c0b7dd1-d40b-584c-a123-a41640f87eec"
|
||
version = "1.0.9+4"
|
||
|
||
[[Xorg_libXcursor_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg", "Xorg_libXfixes_jll", "Xorg_libXrender_jll"]
|
||
git-tree-sha1 = "12e0eb3bc634fa2080c1c37fccf56f7c22989afd"
|
||
uuid = "935fb764-8cf2-53bf-bb30-45bb1f8bf724"
|
||
version = "1.2.0+4"
|
||
|
||
[[Xorg_libXdmcp_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
|
||
git-tree-sha1 = "4fe47bd2247248125c428978740e18a681372dd4"
|
||
uuid = "a3789734-cfe1-5b06-b2d0-1dd0d9d62d05"
|
||
version = "1.1.3+4"
|
||
|
||
[[Xorg_libXext_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg", "Xorg_libX11_jll"]
|
||
git-tree-sha1 = "b7c0aa8c376b31e4852b360222848637f481f8c3"
|
||
uuid = "1082639a-0dae-5f34-9b06-72781eeb8cb3"
|
||
version = "1.3.4+4"
|
||
|
||
[[Xorg_libXfixes_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg", "Xorg_libX11_jll"]
|
||
git-tree-sha1 = "0e0dc7431e7a0587559f9294aeec269471c991a4"
|
||
uuid = "d091e8ba-531a-589c-9de9-94069b037ed8"
|
||
version = "5.0.3+4"
|
||
|
||
[[Xorg_libXi_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg", "Xorg_libXext_jll", "Xorg_libXfixes_jll"]
|
||
git-tree-sha1 = "89b52bc2160aadc84d707093930ef0bffa641246"
|
||
uuid = "a51aa0fd-4e3c-5386-b890-e753decda492"
|
||
version = "1.7.10+4"
|
||
|
||
[[Xorg_libXinerama_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg", "Xorg_libXext_jll"]
|
||
git-tree-sha1 = "26be8b1c342929259317d8b9f7b53bf2bb73b123"
|
||
uuid = "d1454406-59df-5ea1-beac-c340f2130bc3"
|
||
version = "1.1.4+4"
|
||
|
||
[[Xorg_libXrandr_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg", "Xorg_libXext_jll", "Xorg_libXrender_jll"]
|
||
git-tree-sha1 = "34cea83cb726fb58f325887bf0612c6b3fb17631"
|
||
uuid = "ec84b674-ba8e-5d96-8ba1-2a689ba10484"
|
||
version = "1.5.2+4"
|
||
|
||
[[Xorg_libXrender_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg", "Xorg_libX11_jll"]
|
||
git-tree-sha1 = "19560f30fd49f4d4efbe7002a1037f8c43d43b96"
|
||
uuid = "ea2f1a96-1ddc-540d-b46f-429655e07cfa"
|
||
version = "0.9.10+4"
|
||
|
||
[[Xorg_libpthread_stubs_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
|
||
git-tree-sha1 = "6783737e45d3c59a4a4c4091f5f88cdcf0908cbb"
|
||
uuid = "14d82f49-176c-5ed1-bb49-ad3f5cbd8c74"
|
||
version = "0.1.0+3"
|
||
|
||
[[Xorg_libxcb_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg", "XSLT_jll", "Xorg_libXau_jll", "Xorg_libXdmcp_jll", "Xorg_libpthread_stubs_jll"]
|
||
git-tree-sha1 = "daf17f441228e7a3833846cd048892861cff16d6"
|
||
uuid = "c7cfdc94-dc32-55de-ac96-5a1b8d977c5b"
|
||
version = "1.13.0+3"
|
||
|
||
[[Xorg_libxkbfile_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg", "Xorg_libX11_jll"]
|
||
git-tree-sha1 = "926af861744212db0eb001d9e40b5d16292080b2"
|
||
uuid = "cc61e674-0454-545c-8b26-ed2c68acab7a"
|
||
version = "1.1.0+4"
|
||
|
||
[[Xorg_xcb_util_image_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg", "Xorg_xcb_util_jll"]
|
||
git-tree-sha1 = "0fab0a40349ba1cba2c1da699243396ff8e94b97"
|
||
uuid = "12413925-8142-5f55-bb0e-6d7ca50bb09b"
|
||
version = "0.4.0+1"
|
||
|
||
[[Xorg_xcb_util_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg", "Xorg_libxcb_jll"]
|
||
git-tree-sha1 = "e7fd7b2881fa2eaa72717420894d3938177862d1"
|
||
uuid = "2def613f-5ad1-5310-b15b-b15d46f528f5"
|
||
version = "0.4.0+1"
|
||
|
||
[[Xorg_xcb_util_keysyms_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg", "Xorg_xcb_util_jll"]
|
||
git-tree-sha1 = "d1151e2c45a544f32441a567d1690e701ec89b00"
|
||
uuid = "975044d2-76e6-5fbe-bf08-97ce7c6574c7"
|
||
version = "0.4.0+1"
|
||
|
||
[[Xorg_xcb_util_renderutil_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg", "Xorg_xcb_util_jll"]
|
||
git-tree-sha1 = "dfd7a8f38d4613b6a575253b3174dd991ca6183e"
|
||
uuid = "0d47668e-0667-5a69-a72c-f761630bfb7e"
|
||
version = "0.3.9+1"
|
||
|
||
[[Xorg_xcb_util_wm_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg", "Xorg_xcb_util_jll"]
|
||
git-tree-sha1 = "e78d10aab01a4a154142c5006ed44fd9e8e31b67"
|
||
uuid = "c22f9ab0-d5fe-5066-847c-f4bb1cd4e361"
|
||
version = "0.4.1+1"
|
||
|
||
[[Xorg_xkbcomp_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg", "Xorg_libxkbfile_jll"]
|
||
git-tree-sha1 = "4bcbf660f6c2e714f87e960a171b119d06ee163b"
|
||
uuid = "35661453-b289-5fab-8a00-3d9160c6a3a4"
|
||
version = "1.4.2+4"
|
||
|
||
[[Xorg_xkeyboard_config_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg", "Xorg_xkbcomp_jll"]
|
||
git-tree-sha1 = "5c8424f8a67c3f2209646d4425f3d415fee5931d"
|
||
uuid = "33bec58e-1273-512f-9401-5d533626f822"
|
||
version = "2.27.0+4"
|
||
|
||
[[Xorg_xtrans_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
|
||
git-tree-sha1 = "79c31e7844f6ecf779705fbc12146eb190b7d845"
|
||
uuid = "c5fb5394-a638-5e4d-96e5-b29de1b5cf10"
|
||
version = "1.4.0+3"
|
||
|
||
[[Zlib_jll]]
|
||
deps = ["Libdl"]
|
||
uuid = "83775a58-1f1d-513f-b197-d71354ab007a"
|
||
version = "1.2.12+3"
|
||
|
||
[[Zstd_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
|
||
git-tree-sha1 = "e45044cd873ded54b6a5bac0eb5c971392cf1927"
|
||
uuid = "3161d3a3-bdf6-5164-811a-617609db77b4"
|
||
version = "1.5.2+0"
|
||
|
||
[[fzf_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
|
||
git-tree-sha1 = "868e669ccb12ba16eaf50cb2957ee2ff61261c56"
|
||
uuid = "214eeab7-80f7-51ab-84ad-2988db7cef09"
|
||
version = "0.29.0+0"
|
||
|
||
[[libaom_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
|
||
git-tree-sha1 = "3a2ea60308f0996d26f1e5354e10c24e9ef905d4"
|
||
uuid = "a4ae2306-e953-59d6-aa16-d00cac43593b"
|
||
version = "3.4.0+0"
|
||
|
||
[[libass_jll]]
|
||
deps = ["Artifacts", "Bzip2_jll", "FreeType2_jll", "FriBidi_jll", "HarfBuzz_jll", "JLLWrappers", "Libdl", "Pkg", "Zlib_jll"]
|
||
git-tree-sha1 = "5982a94fcba20f02f42ace44b9894ee2b140fe47"
|
||
uuid = "0ac62f75-1d6f-5e53-bd7c-93b484bb37c0"
|
||
version = "0.15.1+0"
|
||
|
||
[[libblastrampoline_jll]]
|
||
deps = ["Artifacts", "Libdl", "OpenBLAS_jll"]
|
||
uuid = "8e850b90-86db-534c-a0d3-1478176c7d93"
|
||
version = "5.1.1+0"
|
||
|
||
[[libfdk_aac_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
|
||
git-tree-sha1 = "daacc84a041563f965be61859a36e17c4e4fcd55"
|
||
uuid = "f638f0a6-7fb0-5443-88ba-1cc74229b280"
|
||
version = "2.0.2+0"
|
||
|
||
[[libpng_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg", "Zlib_jll"]
|
||
git-tree-sha1 = "94d180a6d2b5e55e447e2d27a29ed04fe79eb30c"
|
||
uuid = "b53b4c65-9356-5827-b1ea-8c7a1a84506f"
|
||
version = "1.6.38+0"
|
||
|
||
[[libvorbis_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Ogg_jll", "Pkg"]
|
||
git-tree-sha1 = "b910cb81ef3fe6e78bf6acee440bda86fd6ae00c"
|
||
uuid = "f27f6e37-5d2b-51aa-960f-b287f2bc3b7a"
|
||
version = "1.3.7+1"
|
||
|
||
[[nghttp2_jll]]
|
||
deps = ["Artifacts", "Libdl"]
|
||
uuid = "8e850ede-7688-5339-a07c-302acd2aaf8d"
|
||
version = "1.48.0+0"
|
||
|
||
[[p7zip_jll]]
|
||
deps = ["Artifacts", "Libdl"]
|
||
uuid = "3f19e933-33d8-53b3-aaab-bd5110c3b7a0"
|
||
version = "17.4.0+0"
|
||
|
||
[[x264_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
|
||
git-tree-sha1 = "4fea590b89e6ec504593146bf8b988b2c00922b2"
|
||
uuid = "1270edf5-f2f9-52d2-97e9-ab00b5d0237a"
|
||
version = "2021.5.5+0"
|
||
|
||
[[x265_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
|
||
git-tree-sha1 = "ee567a171cce03570d77ad3a43e90218e38937a9"
|
||
uuid = "dfaa095f-4041-5dcd-9319-2fabd8486b76"
|
||
version = "3.5.0+0"
|
||
|
||
[[xkbcommon_jll]]
|
||
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg", "Wayland_jll", "Wayland_protocols_jll", "Xorg_libxcb_jll", "Xorg_xkeyboard_config_jll"]
|
||
git-tree-sha1 = "9ebfc140cc56e8c2156a15ceac2f0302e327ac0a"
|
||
uuid = "d8fb68d0-12a3-5cfd-a85a-d49703b185fd"
|
||
version = "1.4.1+0"
|
||
"""
|
||
|
||
# ╔═╡ Cell order:
|
||
# ╟─349d7534-6212-11eb-2bc5-db5be39b6bb6
|
||
# ╠═96ec00fc-6f14-11eb-329e-19e4835643db
|
||
# ╠═6abc4d6a-da75-42bf-a62a-b98bb69585aa
|
||
# ╟─237ef27e-6266-11eb-3cf4-1b2223eabfd9
|
||
# ╠═6c060eec-6266-11eb-0b23-e5be08d78823
|
||
# ╟─830c9ed0-6266-11eb-27ba-07773c842fed
|
||
# ╟─f4270146-6216-11eb-391e-01a476fcfccd
|
||
# ╠═b5fff126-6215-11eb-1018-bd2e4f638f65
|
||
# ╟─b9dbaf62-6215-11eb-3a7d-0b882b1a10b0
|
||
# ╟─3249157e-6267-11eb-3dca-8949d7c0e3c9
|
||
# ╠═ce1d05da-6267-11eb-136c-23c5c54a1559
|
||
# ╟─cf933c04-6267-11eb-3317-ed1a42e8c64e
|
||
# ╟─1695a810-6268-11eb-3932-fb8885097f70
|
||
# ╠═77cefbd4-662e-11eb-1b1d-91da61cc3823
|
||
# ╟─88776120-662e-11eb-1542-fd26e4f126b1
|
||
# ╟─874a1a5c-6632-11eb-2705-e914f01b9762
|
||
# ╠═812bbd7e-6632-11eb-29f8-3f48329f0ac9
|
||
# ╠═aeaa97ae-6632-11eb-0ea2-7febd8b3e965
|
||
# ╟─b0d35a9a-662e-11eb-34f5-c9a5fd9bb9a6
|
||
# ╠═d285737a-662f-11eb-390e-1d1e2437de71
|
||
# ╟─de2a045c-662f-11eb-1d80-65fe7d8e0db3
|
||
# ╟─5d04cbea-6630-11eb-3bee-c182aa912653
|
||
# ╠═0ae0cf56-6632-11eb-262a-191ea74ec517
|
||
# ╟─f279b1ee-6631-11eb-0809-bf0699c636f2
|
||
# ╟─0fe8c31e-663a-11eb-1acb-17d3d7615e64
|
||
# ╠═478dde3c-663a-11eb-3244-e7449c93b3a5
|
||
# ╟─68f2b1b0-663a-11eb-1b6d-b176d905f65b
|
||
# ╠═8d509116-663b-11eb-0e98-dd27598740fe
|
||
# ╟─bb8694b8-663b-11eb-03a1-49713346bdf3
|
||
# ╟─06c9bcec-663d-11eb-3062-85c0983a79eb
|
||
# ╠═9fd96950-6651-11eb-25f7-c964ab504b4a
|
||
# ╟─de2903cc-6652-11eb-30c3-7114b15fa6e1
|
||
# ╟─34824462-6654-11eb-2b38-19d14aa309af
|
||
# ╠═6895356c-6655-11eb-3849-b3fa387df754
|
||
# ╟─6b9a4b64-6656-11eb-10ce-7b4a8b3cd6a4
|
||
# ╟─9687bc24-666c-11eb-3b1e-edb5c448bad8
|
||
# ╟─a4a9afe4-6656-11eb-0664-83cb32ce934b
|
||
# ╟─9d3e9a92-6469-11eb-2952-b37367644c48
|
||
# ╠═f3cd5320-66d6-11eb-191c-4b4d8cba940d
|
||
# ╠═f5805956-66d6-11eb-04e8-b1faae8f0d3c
|
||
# ╠═c72359f4-66d7-11eb-395f-b3a1983a6eea
|
||
# ╟─bdea6774-66d7-11eb-1c62-8f0e935f98ef
|
||
# ╠═fb73ea0c-66d7-11eb-001c-23033aee228a
|
||
# ╟─07491ca8-66d8-11eb-3304-99f911b4bd1d
|
||
# ╟─2b99da2c-666d-11eb-1c64-337654a9d8f2
|
||
# ╠═d39fbca0-66db-11eb-1aae-7b29f559cb01
|
||
# ╟─1bcd317c-66e8-11eb-10df-c132d5f79155
|
||
# ╠═66c4f3fc-66db-11eb-0927-ebe1d40eeb3b
|
||
# ╟─2c63db0a-66dc-11eb-1a45-7902d591c3e1
|
||
# ╟─575d9e52-6468-11eb-2f95-63cd3920f91a
|
||
# ╠═2e6c3c30-66e6-11eb-10f0-ddaa1752cff9
|
||
# ╟─d701d778-66e7-11eb-16c2-ab49fc06e992
|
||
# ╠═055baa32-66e7-11eb-20fc-575565bda51b
|
||
# ╟─6a3905f4-66e6-11eb-0607-5534821caee6
|
||
# ╟─91c222ea-66e6-11eb-28ce-c1f1424525c8
|
||
# ╠═b5db566a-66e6-11eb-35fb-17d3fc4e258c
|
||
# ╟─a44eb7ea-66e9-11eb-10fa-2b0936b9f489
|
||
# ╠═d8f306ca-66e9-11eb-3728-156d0328250b
|
||
# ╟─2561d38a-66ea-11eb-10ab-27db1a87970b
|
||
# ╟─673cc322-666e-11eb-107f-2b9bd6826ad5
|
||
# ╠═1bf921be-66eb-11eb-089a-97dfe9418b32
|
||
# ╟─455d0b7c-66eb-11eb-3167-4b204ac741a5
|
||
# ╟─529a7324-66eb-11eb-0c1f-c37639e37a6e
|
||
# ╠═a87e36c4-66eb-11eb-223e-a1b077dca672
|
||
# ╟─b135affc-66eb-11eb-188b-a32ef1478ee6
|
||
# ╟─0aa99f86-6f97-11eb-2141-2d35c3e0857d
|
||
# ╟─f77039b0-6f97-11eb-177b-2730efcb4dcd
|
||
# ╠═fec108ca-6f97-11eb-06d9-6fe1646f8b98
|
||
# ╟─1559f57e-6f98-11eb-3539-1b1ae82c439b
|
||
# ╟─36e6783c-6f98-11eb-0b09-db56907e370d
|
||
# ╠═3f4422ce-6f98-11eb-111f-4d1624a326c7
|
||
# ╟─44ee1586-6f98-11eb-3452-f7db9e3738ad
|
||
# ╠═4a8f3088-6f98-11eb-1d0e-4b1ba2e676ae
|
||
# ╟─50cc4f32-6f98-11eb-25a4-ebaf581955ea
|
||
# ╠═56177258-6f98-11eb-276f-7d8053bdcb86
|
||
# ╟─5733a026-6f98-11eb-1b50-c75f87fbabe5
|
||
# ╠═5d770eb4-6f98-11eb-3206-8d26f2717981
|
||
# ╟─6824d1f2-6f98-11eb-12f1-adf1271af917
|
||
# ╠═6debc444-6f98-11eb-3c9e-4dc533fe13ec
|
||
# ╟─77d17b00-6f98-11eb-37ad-dd347db13fb3
|
||
# ╟─8b852fd4-6f98-11eb-1b3a-7ff47c51e99e
|
||
# ╠═8f566768-6f98-11eb-20ae-45d6f39cd210
|
||
# ╟─961e9cd2-6f98-11eb-362c-517edab85a8c
|
||
# ╠═a32f2a4a-6f98-11eb-18f9-efb51aac288c
|
||
# ╟─acc530cc-6f98-11eb-330e-077fcaf5bd62
|
||
# ╠═e5ca1bf8-6f98-11eb-1bd9-6f2f1fbe55c9
|
||
# ╟─eba05204-6f98-11eb-1e7c-4f7b49962f23
|
||
# ╠═f22b7874-6f98-11eb-0417-1de0d171c4ad
|
||
# ╟─f8265ba4-6f98-11eb-3938-0b38b2b93285
|
||
# ╠═fd8522ec-6f98-11eb-0a3c-01a91ee48de9
|
||
# ╟─0cce95b2-6f99-11eb-1161-1d446c3bbe44
|
||
# ╠═1a12d5a8-6f99-11eb-0e46-1529f881a3b0
|
||
# ╟─214ce458-6f99-11eb-0963-7965b5fba93a
|
||
# ╠═29efc990-6f99-11eb-0633-63fb74c5bebf
|
||
# ╟─2f3b762e-6f99-11eb-12f0-2d42ed8237e0
|
||
# ╟─372fa56c-6f99-11eb-115d-275699f1c05c
|
||
# ╠═473b6dec-6f99-11eb-04f0-07006f1996ba
|
||
# ╟─bf493588-6f14-11eb-3ddf-b7ce036aff36
|
||
# ╟─00000000-0000-0000-0000-000000000001
|
||
# ╟─00000000-0000-0000-0000-000000000002
|